Selenium is a comprehensive project that provides various tools and dependency packages for web browser automation. Additionally, Selenium provides the infrastructure for the W3C WebDriver specification.
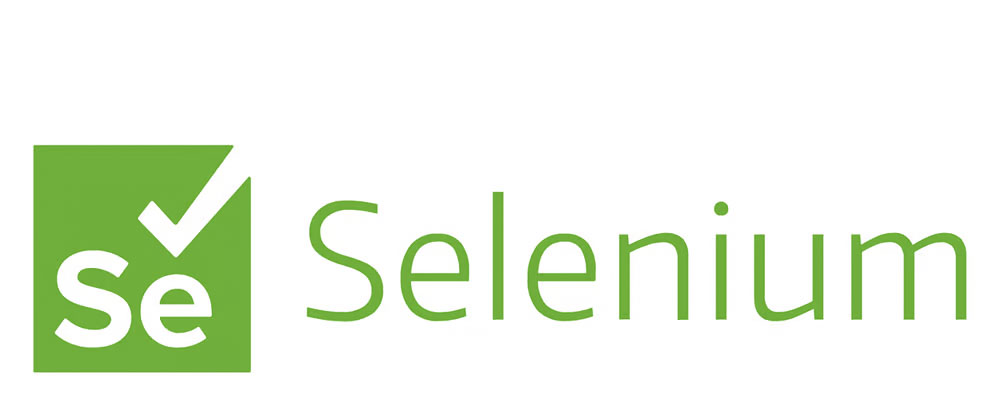
Selenium also supports multiple languages for driving, such as java/python/CSharp/Ruby/JavaScript/Kotlin and other development languages. Today we use python.
Install python
# install python3
yum install python3
# install pip3
yum install python3-pip
# install selenium library
pip3 install selenium
Python script
# Note: please install pip3 and selenium libraries before running
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from concurrent.futures import ThreadPoolExecutor
import time
def login_and_do_stuff(url):
# Set Chrome options
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument('ignore-certificate-errors')
chrome_options.add_argument('--start-maximized') # Maximize the browser window
chrome_options.set_capability("browserName", "chrome")
chrome_options.set_capability("platformName", "Linux")
driver = webdriver.Remote(command_executor=selenium_grid_url, options=chrome_options)
driver.implicitly_wait(10)
try:
driver.get(url)
# Perform login operation
username_input = driver.find_element(By.ID, "username")
password_input = driver.find_element(By.ID, "password")
login_button = driver.find_element(By.ID, "login")
# Enter account password
username_input.send_keys("testuser")
password_input.send_keys("abc-123456")
# Click the login button
login_button.click()
# Wait for five seconds
time.sleep(5)
# Wait for login to complete
WebDriverWait(driver, 10).until(EC.url_changes(url))
# Perform other operations...
# Click the username dropdown to display the Log Off button
username_dropdown = driver.find_element(By.ID, "topbar-username")
username_dropdown.click()
# Wait for the Log Off button to appear
logout_button = WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.ID, "logout")))
logout_button.click()
# Wait for five seconds
time.sleep(5)
# Re-login
driver.get(url)
username_input = driver.find_element(By.ID, "username")
password_input = driver.find_element(By.ID, "password")
login_button = driver.find_element(By.ID, "login")
username_input.send_keys("testuser")
password_input.send_keys("abc-123456")
login_button.click()
# Wait for re-login to complete
WebDriverWait(driver, 10).until(EC.url_changes(url))
# Perform other operations...
finally:
# Do not close the browser here, but rather close it uniformly in the main function
pass
# Close the window
# driver.quit()
# Selenium Grid URL
selenium_grid_url = "http://192.168.100.10/wd/hub"
# The page to open
page_url = 'https://www.test.com/test'
# Number of Windows open at the same time
num_windows = 100
# Open multiple Windows concurrently using thread pools
with ThreadPoolExecutor(max_workers=num_windows) as executor:
futures = [executor.submit(login_and_do_stuff, page_url) for _ in range(num_windows)]
# Wait for all tasks to complete
for future in futures:
future.result()
# Add an appropriate wait here to ensure that the browser has enough time to perform the action
time.sleep(5)
The above script will open the login page and then look for the corresponding element to log in. After successful login, log out and then log in again, a simple case.
Comments NOTHING